Unity C# Beginner Tutorial | How to Calculate Direction & Distance in 3D
In this story, I’m going to show you the idea behind how to get direction in Unity. Every position in 3d space is a vector. So in Unity, Vector3 is the representation of 3D vectors and points thus every gameobject in Unity has its vector position in 3d space.
We can use these vectors to calculate everything in our 3d spaces. In our case, we want to get the direction. For example, if we want to get the direction or distance of the enemy from our position. How can we calculate the direction of CubeB(green) from Cube A(red)?
Related Posts
Idea
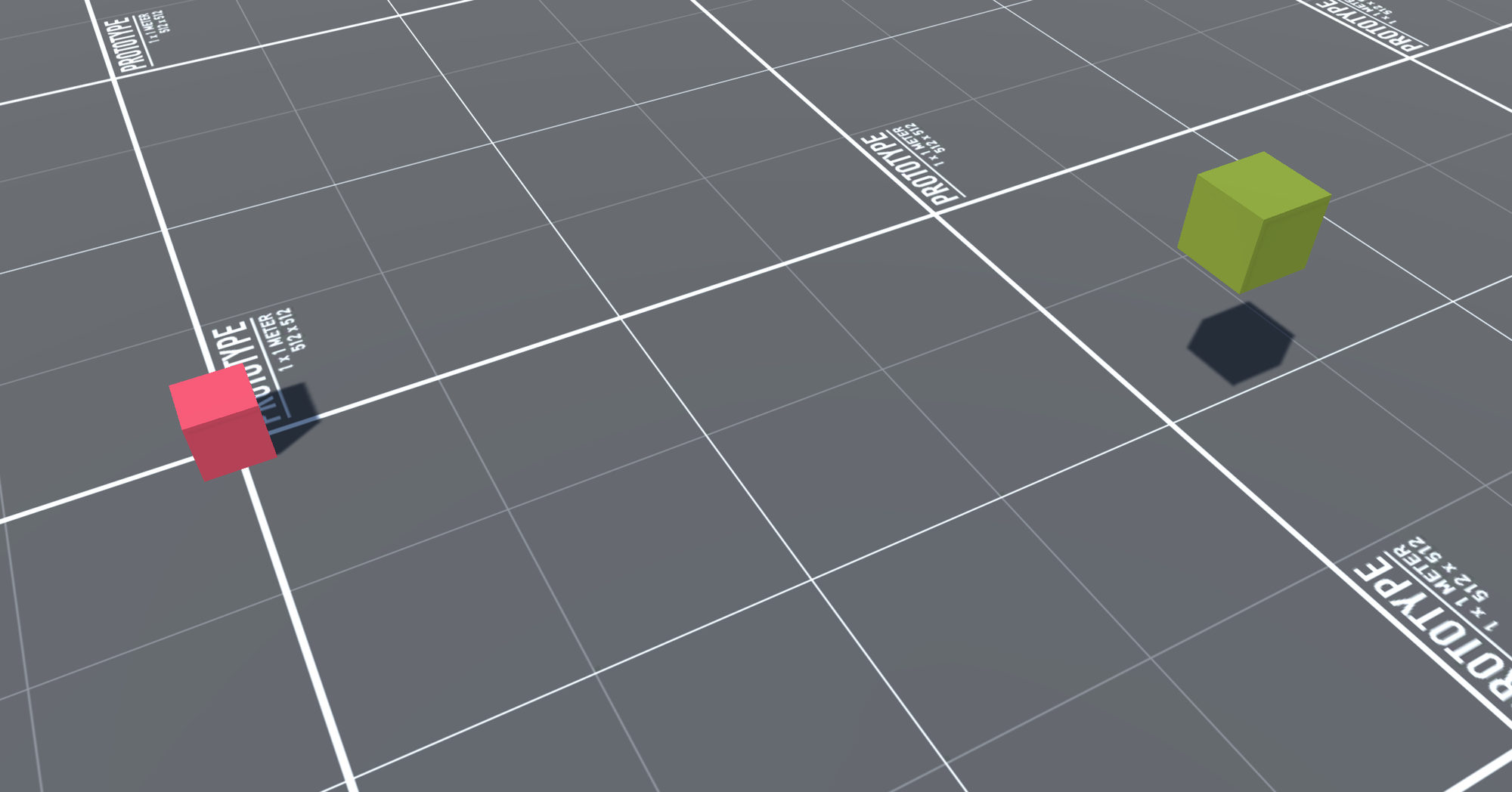
As we know that everything in 3d space has its vector position.
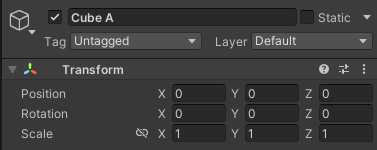
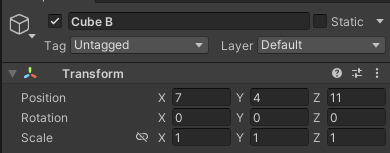
It’s a coordinate system.
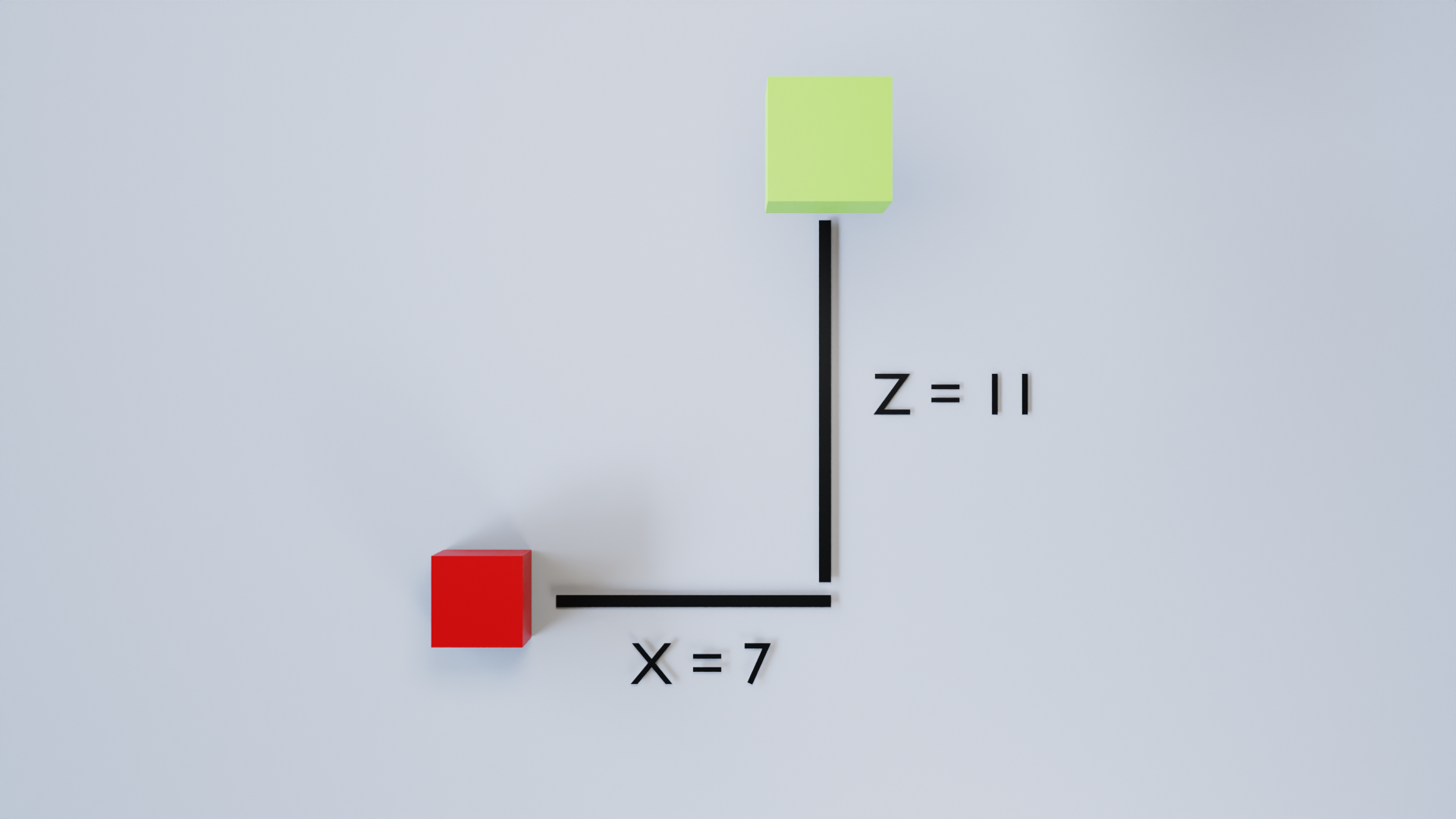
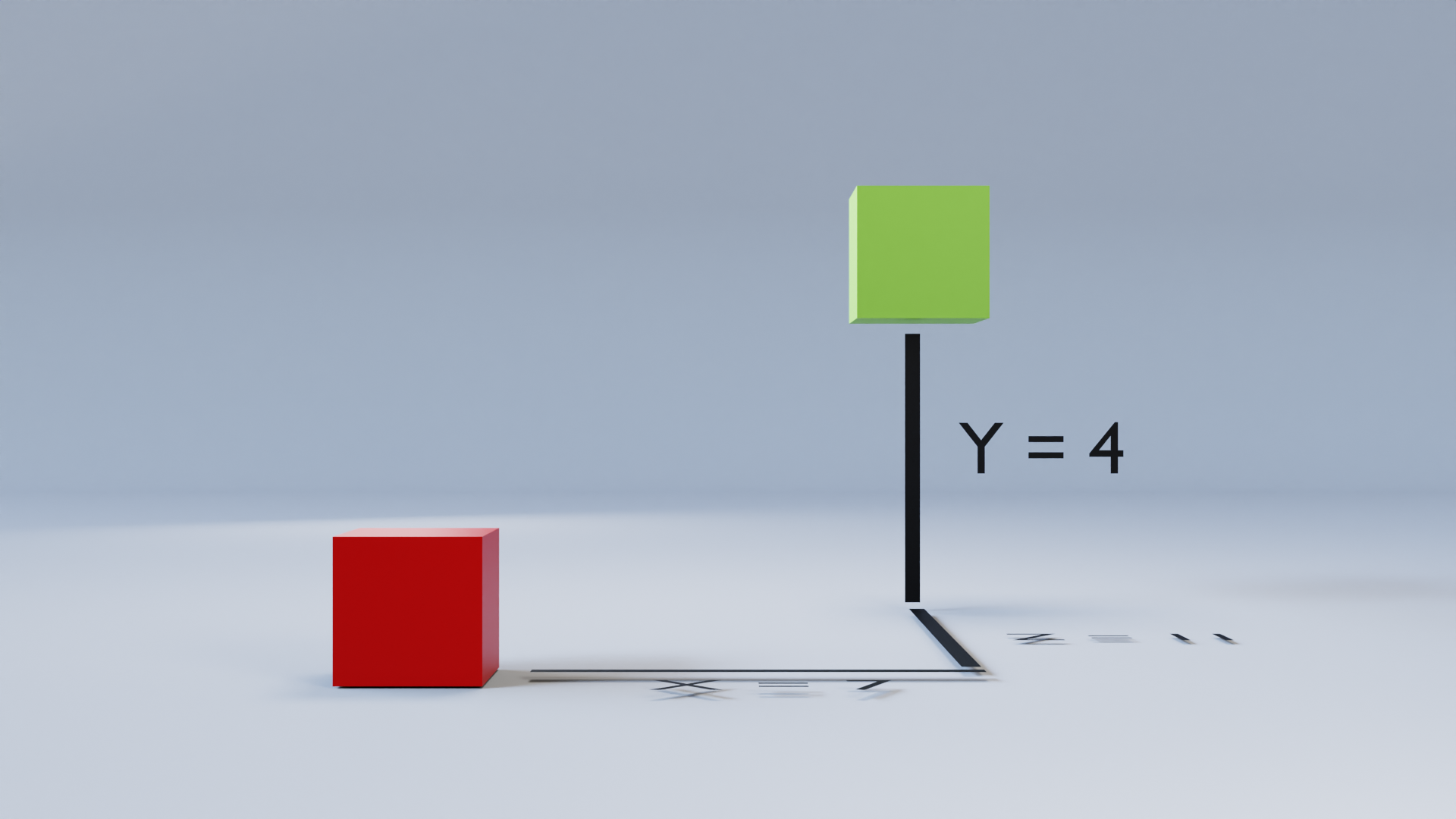
This is how we can get the direction and distance of a vector, it’s Pythagoras Theorem.
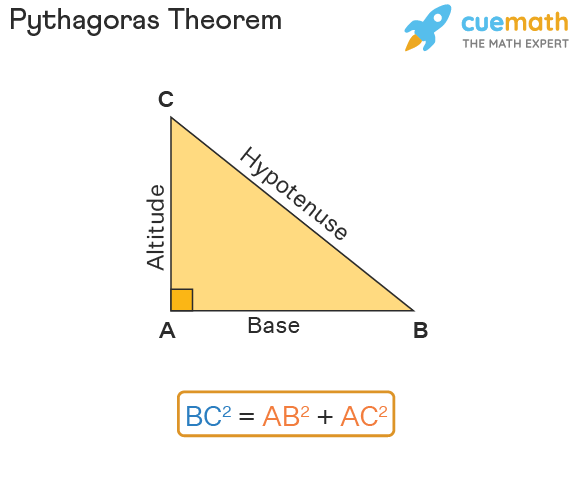
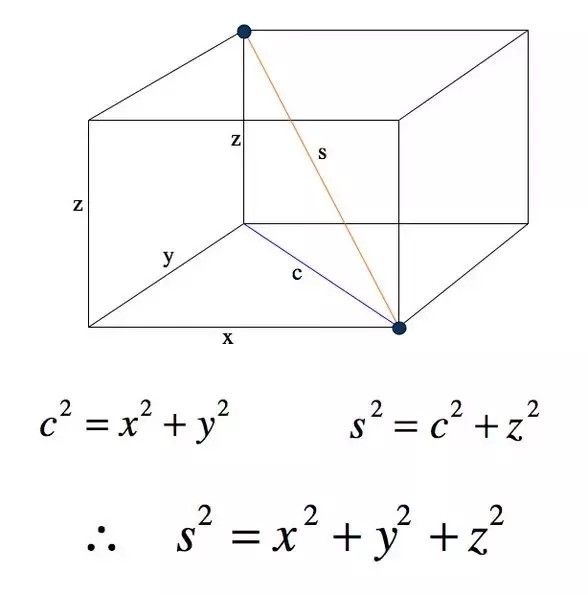
In our case, it should be distance² = 7² + 4² + 11². Then distance = Square root(7² + 4 ² + 11²).
This is just the idea behind the calculation, but in Unity when don't have to calculate everything manually. It’s done by the engine. It’s way easier than that.

Code
So how can we calculate the direction of these two game objects in Unity? The formula is this: Direction = Destination-Source
Here is a simple script to get the direction of two game objects.
Variables
- target: the position of the target gameobject you want to get
Update method
- Vector3 direction: vector3 variable to save the target direction value
- transform.position: the position of this gameobject(the gameobject which you put this script in. )
- Vector3 direction = target.position-transform.position: this is the formula. We subtract the vector position of this gameobject (Cube A) from the target vector position (Cube B) and store the value in the direction variable.
Real situation example
This is also useful if you want to know the distance between a gameobject, or if you want to create an enemy system where it chases the player! In this example, once we have the direction we can make Cube A move to the target.

- transform.Translate(): is a Unity API that Moves the transform in the direction and distance of translation.
- direction.normalized: our Vector3 direction value that we calculated earlier and normalized is to make the distance length 1 but keep the same direction.
- Time.deltaTime: is the interval in seconds from the last frame to the current one. This will make the movement 1 unit per second independent of your machine frame rate.
- transform.Translate(direction.normalized* Time.deltaTime); This whole line is to move Cube A in a direction toward Cube B. Why we normalized the direction is because we want to make the direction length 1 and then we time it with Time.deltaTime. This Time.deltaTime is to make the movement smooth and does not depend on your machine frame rate. Try to remove Time.deltaTime and you’ll see the difference.
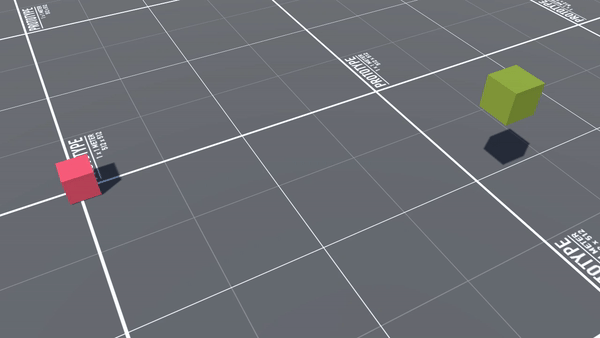
That was that, Cheers!